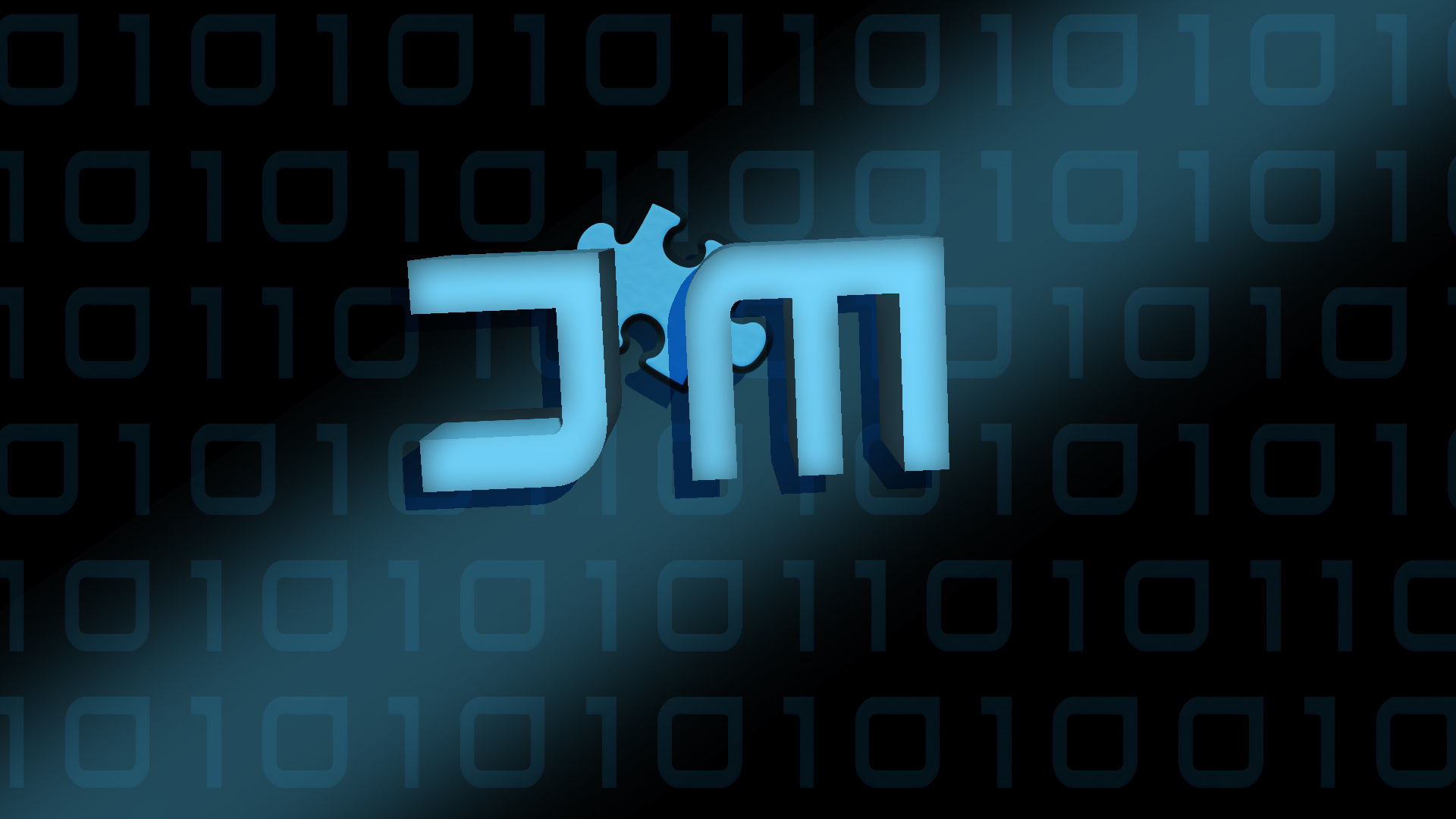
Jamari Marte
C++
Project name: Bank Account (*note condensed sample code)
Description: This program processes different operations of a bank account such as withdrawing, depositing, saving.
Details: This project utilizes functions, classes, inheritance and composition
Main Driver
int main()
{
CheckingAccount jackAccount(1000); // Checking Account object
CheckingAccount lisaAccount(450); // Checking Account object
SavingsAccount samirAccount(9300); // Savings Account object
SavingsAccount ritaAccount(32); // Savings Account object
jackAccount.deposit(1000); // depositing an amount inside of an account
lisaAccount.deposit(2300); // depositing an amount inside of an account
samirAccount.deposit(800); // depositing an amount inside of an account
ritaAccount.deposit(500); // depositing an amount inside of an account
cout << "***********************************" << endl;
jackAccount.print(); // Printing information about an account
lisaAccount.print(); // Printing information about an account
samirAccount.print(); // Printing information about an account
ritaAccount.print(); // Printing information about an account
cout << "***********************************" << endl << endl;
system("pause"); // pausing the system
return 0; // returning 0 back to the operating system
} // end of code
C++ (Continued)
BankAcccount.cpp // base class
#include <iostream>
#include <string>
#include <iomanip>
#include "BankAccount.h"
using namespace std; // Standard Library
// Static variables always have memory allocated
int BankAccount::_acnt = 1000; // static variable used to generate a unique account number
// Default Constructor
BankAccount::BankAccount()
{
_accountId = _acnt++; // setting the accountId to a unique number
_balance = 0; // Initializing balance to zero
}
// Constructor with parameters
BankAccount::BankAccount(double balance)
{
_accountId = _acnt += 100; // Incrementing the account id by 100
_balance = balance; // storing balance inside of balance
}
// Setting the account ID
void BankAccount::setBalance(double x)
{
_balance = x;
}
// Retrieving the balance
double BankAccount::getBalance()
{
return _balance;
}
// Depositing an amount
void BankAccount::deposit(double amount)
{
double newBalance;
newBalance = _balance + amount;
_balance = newBalance;
} // end of code
C++ (Continued)
SavingsAccount.cpp // Derived class
#include <iostream> // iostream header file
#include <string> // string header file
#include<iomanip> // iomanip header file
#include "SavingsAccount.h" // allows us to access members from within the class
using namespace std; // standard library
SavingsAccount::SavingsAccount(double balance)
:BankAccount(balance) // member initializer list
{
_accountId = _acnt; // storing the unique id in accountId
setInterestRate(1.06); // calling the interest rate function
}
// Setting the interest Rate
void SavingsAccount::setInterestRate(double x)
{
_interestRate = x;
}
// Retrieving the interest Rate
double SavingsAccount::getInterestRate()
{
return _interestRate;
}
// Printing information about the savings account
void SavingsAccount::print() const
{
cout << fixed << setprecision(2);
cout << "Savings ACCT#: " << _accountId << " "
<< "Balance: $" << _balance << endl << endl;
}
C++ (Continued)
CheckingAccount.cpp // Derived class
#include <iostream> // iostream header file
#include "CheckingAccount.h" // allows access to the members of the checking account class
using namespace std; // standard library
// constructor with parameters
CheckingAccount::CheckingAccount(double balance)
:BankAccount(balance) // member initializer list
{
_accountId = _acnt; // setting the account id to a unique number
setInterestRate(1.04); // calling the interest rate function and passing in a value
}
// Setting the interest rate
void CheckingAccount::setInterestRate(double x)
{
_interestRate = x;
}
// Retrieving the interestRate
double CheckingAccount::getInterestRate()
{
return _interestRate;
}
// Setting the minimum balance
void CheckingAccount::setBalanceMin(double x)
{
_balanceMin = x;
}
// Retrieving the balance
double CheckingAccount::getBalanceMin()
{
return _balanceMin;
}
// Setting the service charges
void CheckingAccount::setSeviceCharge(double x)
{
_serviceCharge = x;
}
// Retrieving the service charges
double CheckingAccount::getServiceCharge()
{
return _serviceCharge;
} // end of code
Objective - C
Project name: Tic -Tac - Toe w/classes (*note condensed sample code)
Description: A program that allows two users to play a game of tic tac toe against each other
Details: This project utilizes functions, classes, and two-dimensional arrays
Header File
#import <Foundation/Foundation.h>
@interface TicTac : NSObject
{
//Instance variables
char gameBoard[3][3]; // 3X3 array for the gameboard
char playerTurn; // A variable to store the players turn
int row; // A variable to represent the row number
int col; // A variable to represent the column number
int numberOfCellsChosen; // A variable to store the number of cells chosen
BOOL winner; // Flag to indicate who the winner is
}
// Member functions
-(void) beginGame;// This function begins the game
-(void) DisplayGameBoard; // This function displays the game board
-(void)GetInput; // This function gets the users input
-(void)CheckWinner; // This function checks whether player1 has won the game or player2 has won the game
-(void) Play; // This function plays the tictactoe game
@end // end of code
Main Driver
#import <Foundation/Foundation.h>
#import "TicTac.h"
int main(int argc, const char * argv[])
{
@autoreleasepool
{
TicTac *Begin = [[TicTac alloc]init]; // Creating an instance of the class so that it’s member functions can be utilized
[Begin Play]; // Calling the Play Game Method
}
return 0;
} // end of code
Objective - C (continued)
Implementation File
// Invoking all member functions inside of the play function and using the [self] keyword is an example of how you would invoke a function inside of another function
-(void) Play
{
[self beginGame]; //initializing the game
do // Game Loop
{
if (playerTurn == 'X') // if playerTurn is equal to X execute the body
{
[self GetInput]; // function call to get the input
[self CheckWinner]; // function call to check the winner
[self DisplayGameBoard]; // function call to display the game board
playerTurn = 'O'; // switching playerTurn to O
}
else if (playerTurn == 'O') // if playerTurn is equal to O execute the body
{
[self GetInput]; // function call to get the players input
[self CheckWinner]; // function call to check the winner
[self DisplayGameBoard]; // function call to display the gameboard
playerTurn = 'X'; // switching playerTurn to X
}
numberOfCellsChosen++; // updating the number of cells
} while (winner == NO && numberOfCellsChosen < 9);
} // end of code